2장 카카오톡 상품 검출 API는 이미지 콘텐츠를 분석해서 상품들의 위치와 종류를 검출합니다.
필요한 이미지 첨부합니다!
추가적으로 얼굴 이미지를 구하실 분들은 아래 링크에서 이미지를 다운로드하세요!
저작권이 없는 이미지 사이트입니다. --> pixabay.com/
1. REST API
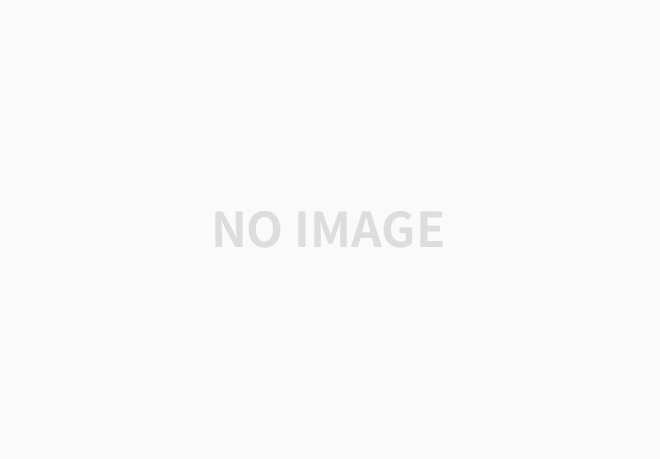
REST API 키를 복사해주세요.
2. 테스트 python 코드
import sys
import argparse
import requests
from PIL import Image, ImageDraw, ImageFont
from io import BytesIO
API_URL = 'https://dapi.kakao.com/v2/vision/product/detect'
MYAPP_KEY = '위 1에서 복사한 RESTAPI키'
def detect_product(filename):
headers = {'Authorization': 'KakaoAK {}'.format(MYAPP_KEY)}
try:
files = { 'image' : open(filename, 'rb')}
resp = requests.post(API_URL, headers=headers, files=files)
resp.status_code
#print(resp.json())
return resp.json()
except Exception as e:
print(str(e))
sys.exit(0)
def show_products(filename, detection_result):
try:
image = Image.open(filename)
except Exception as e:
print(str(e))
sys.exit(0)
draw = ImageDraw.Draw(image)
for obj in detection_result['result']['objects']:
x1 = int(obj['x1']*image.width)
y1 = int(obj['y1']*image.height)
x2 = int(obj['x2']*image.width)
y2 = int(obj['y2']*image.height)
draw.rectangle([(x1,y1), (x2, y2)], fill=None, outline=(255,0,0,255))
draw.text((x1+5,y1+5), obj['class'], (255,0,0))
del draw
return image
if __name__ == "__main__":
parser = argparse.ArgumentParser(description='상품 인식')
#1.parser.add_argument('image_file', type=str, nargs='?',default="./kakao_test/Image/shop4.jpg",help='image url to show product\'s rect')
#2.parser.add_argument('image_file', type=str, nargs='?',default="./shop4.jpg",help='image url to show product\'s rect')
args = parser.parse_args()
detection_result = detect_product(args.image_file)
image = show_products(args.image_file, detection_result)
image.show()
※ 간단한 코드 설명
parser 부분인 얼굴 검출 편에서 설명했으므로 건너가도록 하겠습니다.
경로에 익숙하신 분은 #1.처럼 별도의 이미지 디렉토리를 생성하셔서 경로를 주시면 되고 익숙하지 않으신 분은 python 코드를 작업하는 디렉토리에 이미지를 넣어주시고 #2. 번을 사용해주세요. 둘 중 하나만 사용하셔야 합니다!
* detect_product 함수
기존 코드는 얼굴 검출편과 동일하고 요청에 성공하면 응답은 JSON 객체로 검출된 상품 영역 정보를 받게 됩니다.
#print(resp.json()) 주석 처리된 부분을 해제하시고 어떻게 출력되는지 확인해보세요.
얼굴 검출편과는 다르게 상품의 정보가 나오게 됩니다.
아래와 같이 딕셔너리에서 class부분을 살펴보시면 상품이 무엇인지를 확인할 수 있습니다.
{'width': 1394, 'objects': [{'y2': 0.998958, 'x2': 0.66858, 'score': 0.994, 'y1': 0.746875, 'x1': 0.243902, 'class': 'pants'} |
* show_products 함수
이미지 파일을 읽어서 상품이 있는 위치에 박스와 이름을 만들어주는 함수입니다.
draw.rectangle([(x1, y1), (x2, y2)], fill=None, outline=(255,0,0,255)) # 빨간색으로 박스를 만들고
draw.text((x1+5,y1+5), obj['class'], (255,0,0)) # 빨간색으로 상품 이름을 적어줍니다.
3. 결과 이미지
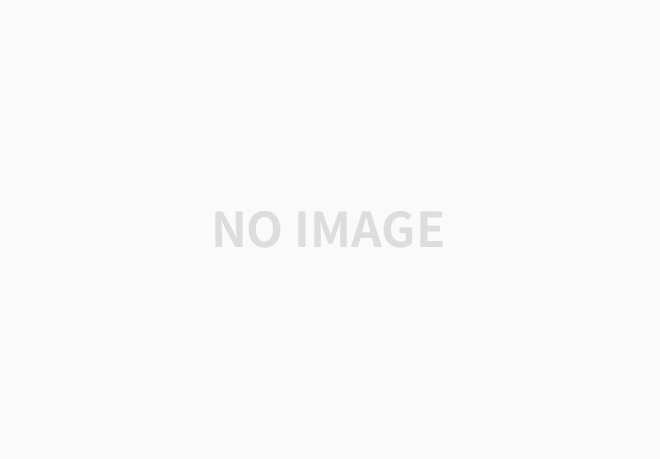
4. 이미지 저장 코드
import sys
import argparse
import requests
from PIL import Image, ImageDraw, ImageFont
from io import BytesIO
import glob
import os
API_URL = 'https://dapi.kakao.com/v2/vision/product/detect'
MYAPP_KEY = '위 1에서 복사한 RESTAPI키'
def detect_product(filename):
headers = {'Authorization': 'KakaoAK {}'.format(MYAPP_KEY)}
try:
files = { 'image' : open(filename, 'rb')}
resp = requests.post(API_URL, headers=headers, files=files)
resp.status_code
#print(resp.json())
return resp.json()
except Exception as e:
print(str(e))
sys.exit(0)
def show_products(filename, detection_result):
try:
image = Image.open(filename)
except Exception as e:
print(str(e))
sys.exit(0)
draw = ImageDraw.Draw(image)
for obj in detection_result['result']['objects']:
x1 = int(obj['x1']*image.width)
y1 = int(obj['y1']*image.height)
x2 = int(obj['x2']*image.width)
y2 = int(obj['y2']*image.height)
draw.rectangle([(x1,y1), (x2, y2)], fill=None, outline=(255,0,0,255))
draw.text((x1+5,y1+5), obj['class'], (255,0,0))
del draw
return image
if __name__ == "__main__":
#1.files=glob.glob('./kakao_test/Image/*.jpg')+glob.glob('./kakao_test/Image/*.jpeg')+glob.glob('./kakao_test/Image/*.png')
#2.files=glob.glob('./*.jpg')+glob.glob('./*.jpeg')+glob.glob('./*.png')
for i in files:
head,tail = os.path.split(i)
detection_result = detect_product(i)
image = show_products(i, detection_result)
image.save(head+'/product_'+tail,'JPEG')
files객체를 생성하실 때 경로에 익숙하신 분은 #1. 번에서 별도의 이미지 디렉토리를 이용하시고 익숙하지 않으신 분은 #2. 번익숙하지 않으신 분은 python 코드를 작업하는 디렉토리에 이미지를 넣어주시고 #2. 번을 사용해주세요. 둘 중 하나만 사용하셔야 합니다!
이후 코드는 1-2. 얼굴 검출[저장편]과 동일하기 때문에 생략하겠습니다!
5. 결과 이미지
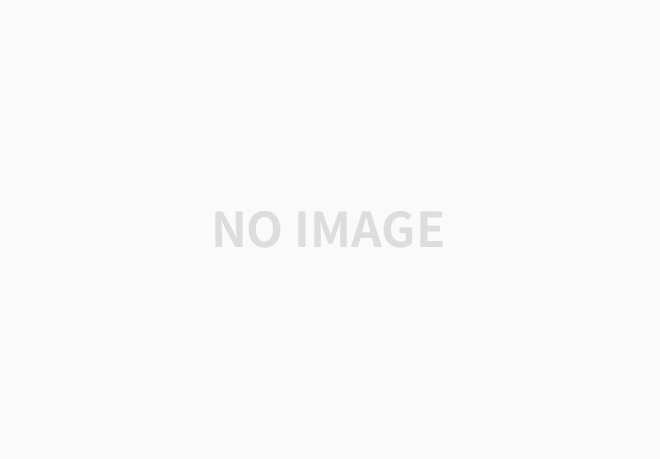
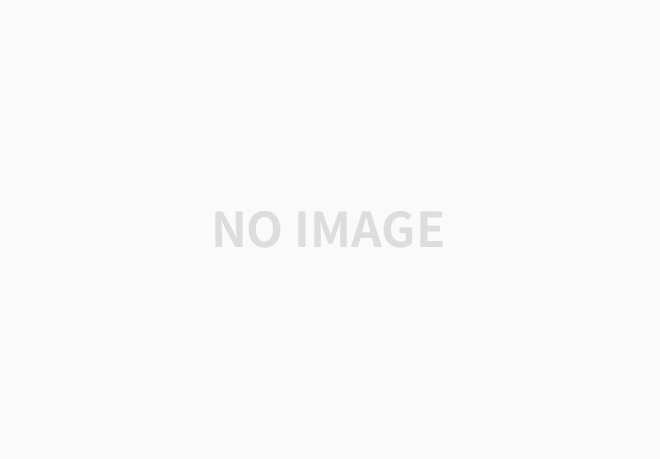
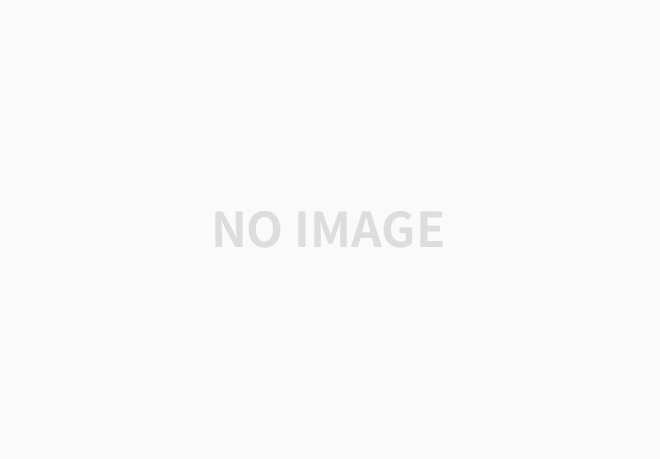
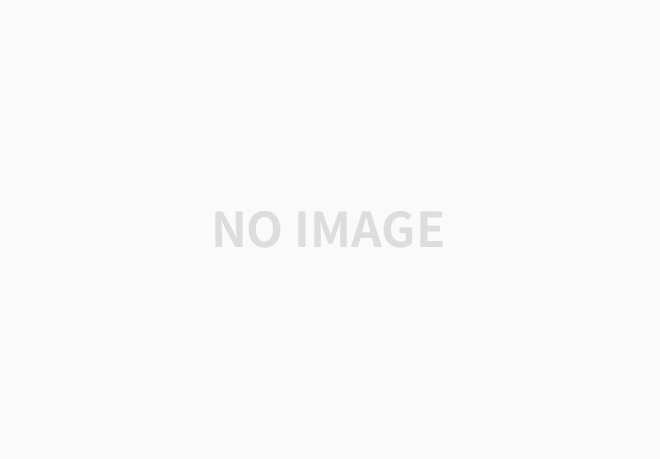
얼굴 검출편과 코드가 대부분 동일해서 설명을 간략하게 하고 넘어갔는데 혹시라도 이해가 안가시는 부분이 있으시다면 댓글에 남겨주세요.
모르시는 부분을 댓글에 남겨주시는 것은 다른 분들에게도 도움이 됩니다. 도와주세용~!
'카카오 API' 카테고리의 다른 글
[python] 10분 만에 카카오톡 비전 사용편 - (3. 썸네일) (2) | 2021.03.10 |
---|---|
[python] 10분 만에 카카오톡 비전 사용편 - (1-2. 얼굴 검출[저장편]) (0) | 2021.03.08 |
[python] 10분 만에 카카오톡 비전 사용편 - (1-1. 얼굴 검출) (0) | 2021.03.05 |
[Python] 10분 만에 카카오톡 메시지 보내기 친구편 - (2. 메시지 보내기) (43) | 2021.01.04 |
[Python] 10분 만에 카카오톡 메시지 보내기 친구편 - (1. 설정 & 사용자 토큰 발급) (25) | 2020.12.31 |